Seed Roles And Users To An Existing Database In ASP.NET MVC 5 Using Identity
Hello,
In this tutorial, I will demonstrate seeding roles and users to an existing database in an ASP.NET MVC 5 application using Identity framework. For simplicity, I will use a Northwind. This database doesn't have membership tables at all.
To begin with, accomplish the steps below:
1. Create an ASP.NET MVC application and then change web.config connectionStrings element to connect to an existing database (Northwind as my example).
2. Under Package Manager Console type
PM> Enable-Migrations
3. Under Package Manager Console type
PM> Add-Migration ASPMembership
* This will create a file Timespan_ASPMembership.cs inside Migrations folder with scripts to create Membership Tables such as AspNetUsers, AspNetRoles and etc.
4. Under Package Manager Console type
PM> Update-Database
* This will add membership tables to Northwind database.
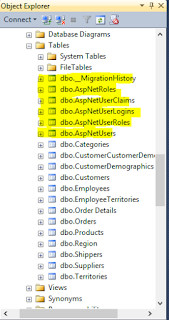
5. To seed Roles and Users, create a class SeedRolesAndUsers.cs inside Models folder. This class references Microsoft.AspNet.Identity and Microsoft.AspNet.Identity.EntityFramework namespaces. The code below adds Customer or Manager role if they didn't exist. And then the remaining part of the code, creates a user assigned to a specific role.
6. In Global.asax.cs, call the seed method and then pass an ApplicationDBContext object to it's parameter.
7. Run the application. You should see the tables AspNetRoles, AspNetUsers, AspNetUserRoles populated with data.
VB.NET Version of this tutorial: Seed Roles and Users to an existing database in ASP.NET MVC 5 using Identity
In this tutorial, I will demonstrate seeding roles and users to an existing database in an ASP.NET MVC 5 application using Identity framework. For simplicity, I will use a Northwind. This database doesn't have membership tables at all.
To begin with, accomplish the steps below:
1. Create an ASP.NET MVC application and then change web.config connectionStrings element to connect to an existing database (Northwind as my example).
2. Under Package Manager Console type
PM> Enable-Migrations
3. Under Package Manager Console type
PM> Add-Migration ASPMembership
* This will create a file Timespan_ASPMembership.cs inside Migrations folder with scripts to create Membership Tables such as AspNetUsers, AspNetRoles and etc.
4. Under Package Manager Console type
PM> Update-Database
* This will add membership tables to Northwind database.
5. To seed Roles and Users, create a class SeedRolesAndUsers.cs inside Models folder. This class references Microsoft.AspNet.Identity and Microsoft.AspNet.Identity.EntityFramework namespaces. The code below adds Customer or Manager role if they didn't exist. And then the remaining part of the code, creates a user assigned to a specific role.
public class SeedRolesAndUsers { protected static void Seed(ApplicationDbContext context) { var userManager = new UserManager<ApplicationUser>(new UserStore<ApplicationUser>(context)); var roleManager = new RoleManager<IdentityRole>(new RoleStore<IdentityRole>(context)); if (!roleManager.RoleExists("Customer")) { var roleresult = roleManager.Create(new IdentityRole("Customer")); } if (!roleManager.RoleExists("Manager")) { var roleresult = roleManager.Create(new IdentityRole("Manager")); } string userName = "jimmymgr01"; string password = "jimmymgr01"; ApplicationUser user = userManager.FindByName(userName); if (user == null) { user = new ApplicationUser() { UserName = userName }; IdentityResult userResult = userManager.Create(user, password); if (userResult.Succeeded) { var result = userManager.AddToRole(user.Id, "Manager"); } } } }
protected void Application_Start() { AreaRegistration.RegisterAllAreas(); FilterConfig.RegisterGlobalFilters(GlobalFilters.Filters); RouteConfig.RegisterRoutes(RouteTable.Routes); BundleConfig.RegisterBundles(BundleTable.Bundles); //call seed method ApplicationDbContext context = new ApplicationDbContext(); SeedRolesAndUsers.Seed(context); }
VB.NET Version of this tutorial: Seed Roles and Users to an existing database in ASP.NET MVC 5 using Identity
Comments
Post a Comment